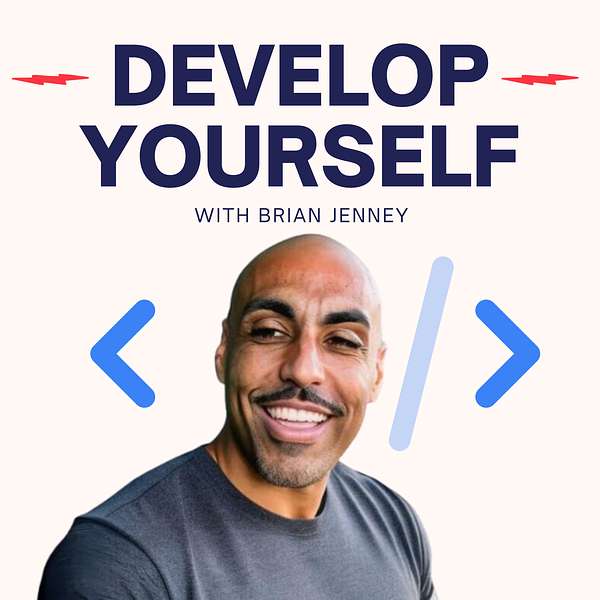
Develop Yourself
To change careers and land your first job as a Software Engineer, you need more than just great software development skills - you need to develop yourself.
Welcome to the podcast that helps you develop your skills, your habits, your network and more, all in hopes of becoming a thriving Software Engineer.
Develop Yourself
#220 - Real-World JavaScript: Why Loops and Arrays Are the Backbone of the Internet
Send a text and I may answer it on next episode (I cannot reply from this service 😢)
You’re learning about JavaScript arrays all wrong.
For loops, zero-indexing, blah blah blah.
But WHY? What makes arrays so damn important?
Let's break down why arrays and objects are the backbone of the internet and a couple common problems that JS beginners should work towards solving.
A video on using APIs with JavaScript: https://youtu.be/4naH4qczQCg
Shameless Plugs
🧠 (NEW) Parsity's The Inner Circle Program - a highly customized roadmap to take you from 0 to hired. For career changers who want to pivot into software.
💼 Zubin's LinkedIn (ex-lawyer, former Google, Brian-look-a-like)
👂🏻Easier Said Than Done Podcast
Already a developer? Check out 👉 Not Another Course
Serious about joining Parsity? Schedule a call with me ☎️
Welcome to the Develop Yourself podcast, where we teach you everything you need to land your first job as a software developer by learning to develop yourself, your skills, your network and more. I'm Brian, your host. So if you're a complete code noob meaning you're just learning how to write code you're maybe encountering JavaScript, and now you've reached the first hill on the mountain that is JavaScript. First of all, let me tell you something you cannot learn JavaScript in 30 days. You cannot learn it in six months. You will not really learn it in a year. I have been learning JavaScript for somewhere around 11 years, now maybe going on 12 years. I'm still learning it. It's a language. It evolves. Imagine speaking English in 1200. Now imagine speaking to a Gen Z. Now imagine speaking to whatever the hell the generation of kids is talking about today Skibbity-toilet and all this weird stuff. Language changes. It evolves. Coding languages are no different. They evolve. Standards change, practices change, norms change. So therefore, once you learn a programming language, you basically will be learning it throughout time, until the day you expire, because you'll probably expire before JavaScript expires.
Speaker 1:Now, as you're learning JavaScript, you're probably encountering your first real hurdle, which is probably gonna be arrays. Why arrays? They're kind of conceptual and most people are learning them all wrong. So what I want to do today is break down two common problems you're going to encounter when working with arrays, their real world application and how to solve them. But first off, what the hell are arrays? So, in JavaScript, an array is a special type of object used to store multiple values in a single variable. It's zero based meaning I'm a human, I count like a human One, two, three, four. Javascript, like most programming languages, uses zero based indexing Fancy way of saying we start counting from zero. So this is like, basically, a list. You can think of an array as a list and you can have multiple values in the list. Great Done, right Now you got it. Now you got arrays. Boom, you're set to go. The real problem, though, when people learn about arrays is they're like well, what's the practical application of an array? Let me explain. Arrays are probably the most important object type in JavaScript. The reason why is because most of the data that you encounter on the web is in an array-like form. So think about it Social media posts, e-commerce sites.
Speaker 1:I like to use social media as an example. You're on Instagram. Think of what a typical post has. Is every post different? Not really. They have a few common attributes. Every post on Instagram will have a picture, it will have some likes, it will have a comment section and it will have a description, maybe some hashtags. This is a uniform shape, right? You can think of this being represented in an object, and if you don't know what an object is, go look it up. Now, think of somewhere like Airbnb. When you go to a page on Airbnb and you search for a house in Los Angeles to spend your weekend with your family or whatever, and you get back a list of what A list of houses. Now what do they have? Some similar attributes. Do you see where I'm going with here? Next time you're on the web and you're looking at any list, whether it's on an e-commerce site or a social media site look for the common attributes.
Speaker 1:Now, the way we represent these common attributes is in an object. In JavaScript, an object has properties and it has values. So a property might be an image. In a social media post, the value might be the actual image. A property might be comment. The value might be you're a loser or something like that, right?
Speaker 1:So arrays can store a list of these objects, these similar representative structures which we can use to then flesh out or construct a web page, and we can make really complex things with these. That's why arrays are so important, and when you learn about how HTTP requests work, when, basically, you open up a web page, it makes a request to some server that probably Jeff Bezos owns, or something like that. That server gets the request and then it returns you some files, maybe some JSON. Json is JavaScript Object Notation. For all intents and purposes, you can think of it as a list of objects. So when you go to Instagram or whatever you're on and you make that request, your phone or your computer makes a request to that server farm that Jeff Bezos owns and it returns you that list of objects. Well, the developer who was making that application almost certainly saw that list as an array full of objects and what they did was loop over it and then returned it on the screen, and then they can do all sorts of wonderful things with that.
Speaker 1:This is why arrays and objects are so gosh dang important, and if you're going to study one thing in JavaScript and you really want to get a good hang on it and get really practical, I strongly suggest that once you move past arrays, objects, fl statements and for loops, you immediately try to start working with APIs. Make some requests to get some data over the web using HTTP protocol Very fancy way for saying make a request over the web to a server somewhere and get some data back. There are tons of free APIs out there. There's a couple fake APIs out there that you can use for testing. Basically, people made these APIs just for people like you that are early on in your journey and want to test out how to use these things and don't want to have to do a bunch of weird setup. It's really simple to do it, and I'll even have a video in the show notes that will walk you through a practical way of how to get started using APIs in JavaScript.
Speaker 1:Now that you know why they're essential, I'm going to break down two problems that are really representative of problems that you will encounter in the real world, or at least encounter if you're doing some stupid interview, because people love to ask these questions in interviews. So let's get to it. Something like a frequency counter is a really common problem. Imagine back to the social media post. Right? So you're looking at a social media post about how much JavaScript sucks and it has a bunch of reactions and the reactions are in an array and you have like love, like wow, like love hate, whatever, whatever, whatever the reactions might have like four different unique types and you might see one of those types represented many times and one type represented fewer times. So, for the example I just gave, we had like three or four times in there. We had love one, you know, two times, we had wow one time. So the problem might be in this case how do you count how many times each reaction?
Speaker 1:What you could do in this scenario is you could loop through the array and if you don't know how to write a for loop, again you got to look it up, because this kind of show is not the greatest way to explain how for loops work, because you really need to visualize this. But what you would do is you'd loop through and then you'd keep track of how many times each particular value occurred using an object and in that object you would look hey, is this a value that I haven't encountered yet? Oh, it is. Well then let me add it to the object and let me increment the counter to one. Let me just set the value of that reaction to one. So if we're looping over this array and we see the value like is the first thing in the array we see, we'd say, cool, let's look in our object. That has all our accounts. Do we have a property called like in here yet? No, we don't. Cool, we'll set it to one. If we see the word like, again, we'll check in our object and say, hey, do we have that particular value? Oh, we do. Well, cool, let's add one to it. And then so on and so forth. So why does this matter? Why is this exercise important? And, by the way, you could dump this into chat GPT and you could say hey, give me an array full of values where I see certain values overrepresented or underrepresented or whatever.
Speaker 1:We have a test like this at Parsity that we used to give out to people to see if they could code their way out of a paper bag. Essentially, and the reason why this is so important E-commerce analytics. How many times does a specific error occur in a log, maybe? How many items do you have in your shopping cart, maybe? What are the number of likes versus dislikes on a YouTube video? These are incredibly important things and even though what I gave you is a fairly trivial example. At some point something like that is happening Somebody, some developer, is going through an array or some massive log full of like values and counting the frequency of those values, and similar to this one.
Speaker 1:There's another problem that comes up a lot, whether in interviews or just in like real life finding the highest and the lowest values. This is another problem. We used to give out at Parsity and, by the way, we've gotten rid of our entrance exam because now we have a conversation with everybody that wants to join, make sure they're the right fit, and we have a very custom curriculum so we can have people that come in at different levels. Now we don't have to have this really wild entrance exam that a lot of people just couldn't pass. If I'm being very honest with you, it's kind of shocking how many people could not pass the entrance exam, even though they claim to have been coding for months.
Speaker 1:So here's another one where you could ask ChatGPT hey, give me an array full of numbers, right, like give me an array full of 10 numbers between one and 50. And the problem that you wanna solve is find the highest and find the lowest. This sounds simple because, as a human, you know how to do this. So how do you do this? Well, you would have to loop through the array and keep track of the current highest and the current lowest. This is what you might even call a greedy approach, which is a common way to approach a coding problem or an algorithm.
Speaker 1:Greedy approach is to say what is the best option I have at the given point. So if you're looking at the very first value in an array, then that's the highest, because at that point you're being greedy. You say, well, what's the best I can get? Oh well, I'm at the first value in an array, then that's the highest, because at that point you're being greedy. You say, well, what's the best I can get? Oh well, am I at the first value in the array? Well, that's the best, because I have nothing to compare it to. And then you say, okay, what about the next one? Is that bigger or lower than my current one? If it's bigger, then I'm switching out my current choice and I'm grabbing that one. Then you compare it to the next one and you do the same thing.
Speaker 1:Think of a greedy billionaire. They don't exist, of course, but just imagine if they did. Think of a greedy billionaire, the mythical greedy billionaire that no one ever heard of, and they would be going here and saying, what's the best dang deal, can I get it? Every step of this array. And they're grabbing that value and they're looking at the next value and saying, oh, I can get more, give me that one. Then they reach the end of the array and they say, okay, whatever I have now is the biggest value I have. Whatever that biggest value I found is the biggest one. And boom, I found the largest value in the array. Now you can do the exact same thing with the lowest. Maybe you're the greedy billionaire and you're looking for the lowest price on labor or something like that, something evil that only an evil billionaire would do. And so you look at the first one, and at the first one, well, you say, well, that's the best I can get because I have nothing to compare it to. Then you look at the next one and you do the same thing Get it. You kind of see how this is working out now. So this isn't just some stupid mathematical exercise or anything like that. Think about finances finding the highest and lowest stock prices. Think about gaming, if you're identifying the top or the lowest score on a leaderboard.
Speaker 1:Back to the e-commerce app. You want to show the most expensive and the least expensive items in a category, and here at a really high level. Here's how you just approach problems like this One. You understand the problem. What is the array representing? What is the question asking you to find? Second, break it down. Use small, simple steps to manipulate the data and track what you need. And, lastly, keep it flexible.
Speaker 1:There are patterns for all this stuff. When you encounter a problem and you're looking to solve it, once you solve it and bang your head against the computer or whatever for a while maybe don't bang your head against your computer, but once you kind of get stuck for a while. Getting stuck is really important. You want to always reach the limit of your technical depth, because if you're too quick to go to ChatGPT one, the memory won't stick and you'll fool yourself into believing that you've actually learned something when you really haven't. So you'll need to bang your head a little bit against your keyboard before you just go on to ChatGPT. But once you do find out, what pattern exists for doing these things the two problems I just talked about they have many patterns that can be used to approach them. You don't have to reinvent the wheel. So these are better than just beginner exercises. These will give you some good foundational skills that'll show up in different scenarios in the real world or at least in your freaking coding.
Speaker 1:Interview Now. If you want to learn JavaScript building in public, jumpstart your career as interview Now. If you want to learn JavaScript building in public, jumpstart your career as a software developer. We have a program called Dev30. It's 99 bucks. It's wildly inexpensive and it's the best program I can think of to not just learn JavaScript but how to start building a network and learning in public. And the best part is it's not a course. It is working for one month with me and some other people in a Slack group and a director of engineering Really excellent deal.
Speaker 1:We start enrolling now and we will launch in April. I hope to see you there and, as usual, hope that's helpful. See ya, that'll do it for today's episode of the Develop Yourself podcast. If you're serious about switching careers and becoming a software developer and building complex software and want to work directly with me and my team, go to parsityio. And complex software and want to work directly with me and my team, go to parsityio. And if you want more information, feel free to schedule a chat by just clicking the link in the show notes. See you next week.